SSG based apps
SSG describes the process of building websites that render at build time. The output is an HTML file, assets such as JavaScript and CSS, and a few other static files.
Introduction
Static site generation is the process of building pages (pre-rendering) into static assets and serving them to users instead of doing it per request, especially when our data is static or doesn't change often. We can also create as many builds as we want, and most of this work can be done on a hosted server, making the process easy. There are many static site generation tools based on React.js, with some of the most interesting ones being Gatsby, Next.js, and Remix, Eleventy. For example, Gatsby has a great ecosystem of plugins to get you started quickly. Next.js is flexible, allowing you to opt into SSG on a per-page basis. Remix, while it doesn't currently support SSG in the traditional sense, strives for page performance through caching assets on edge servers.
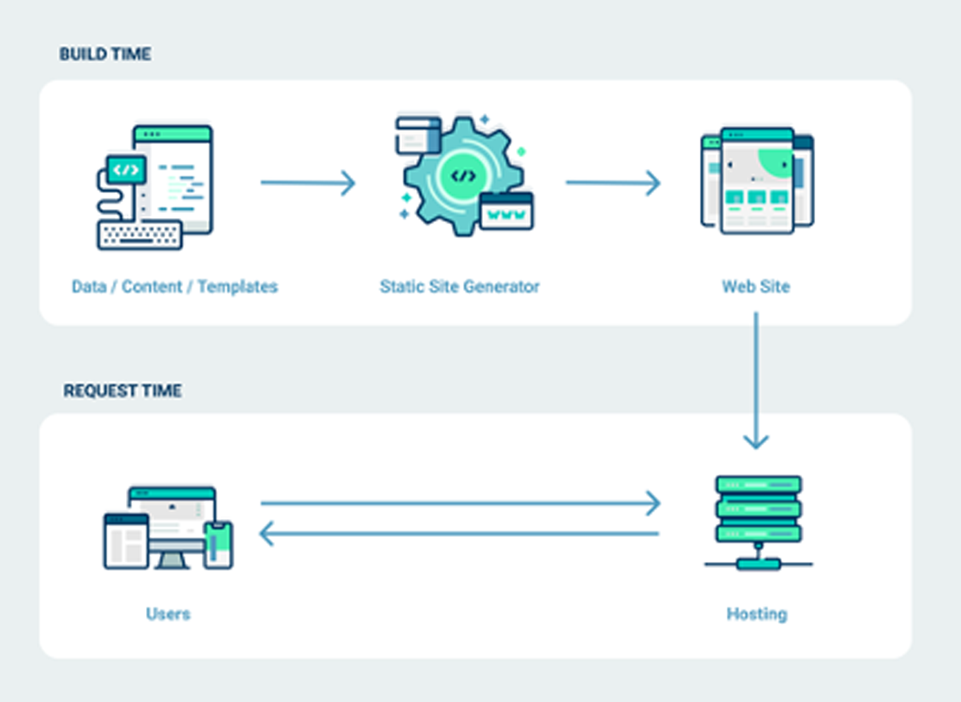
SSG Details
By and large, most popular generators offer a broad range of features that make your work easier, including:
- Support for Markdown language: Most generators don't include full-blown text editors. Instead, they use Markdown language, sometimes even including additional custom Markdown syntaxes.
- Built-in layout and templating options: Designing and styling a website is often the most challenging building process. That's why generators tend to include built-in templating and layout options.
- Support for multiple types of content: Depending on which generator you use, it should support various types of content, such as blog posts, pages, galleries, and more.
- Built-in SEO functionality: No modern website is ready without proper search engine optimization (SEO). If you're considering — or already using — a generator that doesn't offer SEO features or add-ons, you're better off moving to a new tool.
Pros & Cons
- Pros
- SEO , search engine optimisation is one of the top benefits of doing SSG as it make indexing the pages easy for the crawlers.
- Performance : As you can guess, serving an HTML page is much faster for the end-user because the browser doesn't have to do much processing upfront. The pre-rendering makes it easy for the browser to fetch the HTML and render it straight up.
- Security : No site is perfectly secure, but static assets offer very few attack vectors. There is no database to hack and no application server to compromise. By running at the edge on a CDN, there isn't even a single web server one could gain access to.
- Caching with CDNs : Building HTML pages opens the possibility for CDN caching to show its charm. The pages are stored closer to the user globally and hence can be accessed much faster. Every request doesn't have to wait for the server to render the page, it just receives the page from CDN, saving us compute resources and money.
- Cons
- Few or no pre-built templates: The downside of unlimited customization is that it can take longer to get started. Many static site generators do not come with templates, and developers will have to spend a lot of time building them from scratch at first.
- No user-friendly interface: It is harder for non-developer users to publish content using a static site generator. There is no CMS interface, and working with raw unformatted data may be intimidating for users. In addition, developer support is often necessary for making website updates.
- Reference Links (Cheetsheet, shorthand)
Examples
While you can use SSG in any scenario as long as the page can be rendered at the build time, here are some popular use case patterns for SSG
- Marketing websites
- Blogs and documentation
- Portfolio websites
Code Example Snippets
SSG with Gatsby
Developers write functions in Node.js to fetch data, create pages, and fill them with content. Matching these pages with React components (Gatsby calls these "templates") is how Gatsby knows what to render and when.
Builds can be created on a developer's local machine but also on many SaaS hosting solutions like Gatsby Cloud, Netlify, and others. Once a build is complete, it can then be deployed to a CDN for ultra-fast serving to users.
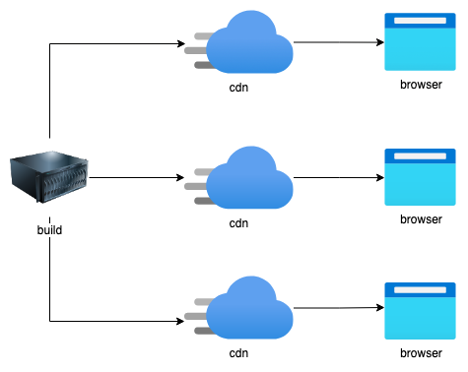
One of the best aspects of Gatsby is that its developer ecosystem is extensive. It has data-source plugins that make it incredibly easy to fetch data from an external API (take Shopify, for example) and image optimization plugins, just to name a few.
example of the code used to generate pages in Gatsby
exports.createPages =asyncfunction({ actions }){
const{ data }=await graphql(`
query {
allMarkdownRemark {
nodes {
fields {
slug
}
}
}
}
`)
data.allMarkdownRemark.forEach(node=\>{
const slug = node.fields.slug
actions.createPage({
path: slug,
component:require.resolve(`./src/templates/blog-post.js`),
context:{ slug: slug },
})
})
};
SSG with Next
There is now a relatively new feature that added SSG support to Next.js, with the unique proposition that it can be done on a per-page basis. The build process is similar to traditional SSG frameworks like Gatsby, but you can choose the pages the SSG occurs on. Developers also have the choice of using incremental static generation (ISR), which is an advanced method that builds pages at runtime if they are not present or need to be rebuilt due to developer-set invalidation.
If you have only chosen to use SSG for your pages, deployment is similar to Gatsby and can be done with a variety of vendors. It uses Node.js to fetch data and build your pages. If you use any of the SSG unsupported features, such as using a server to fetch data and build pages at runtime, you'll have a more involved deployment process.
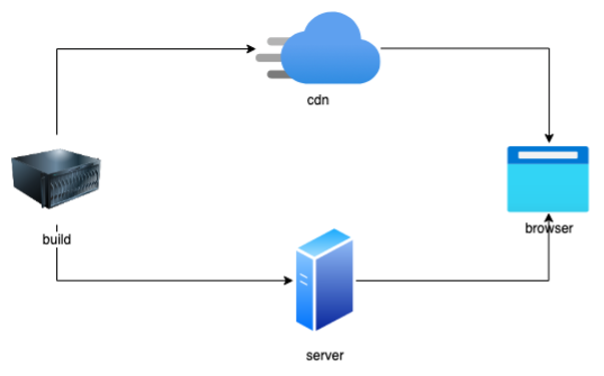
One of the nicest aspects of Next.js is that it gives you the freedom to do what you want. As mentioned, you get the choice on a per-page basis to use SSG, SSR, or the new method, ISR. Better yet, it doesn't prescribe anything like GraphQL when it comes to getting the data into your React components.
Example of the code required for Next.js to statically generate a page
functionBlog({ posts }){
return(
\<ul\>
{posts.map((post)=\>(
\<li\>{post.title}\</li\>
))}
\</ul\>
)
}
exportasyncfunctiongetStaticProps(){
const res =await fetch('\<https://example.com/posts\>')
const posts =await res.json()
return{
props:{
posts,
},
}
}